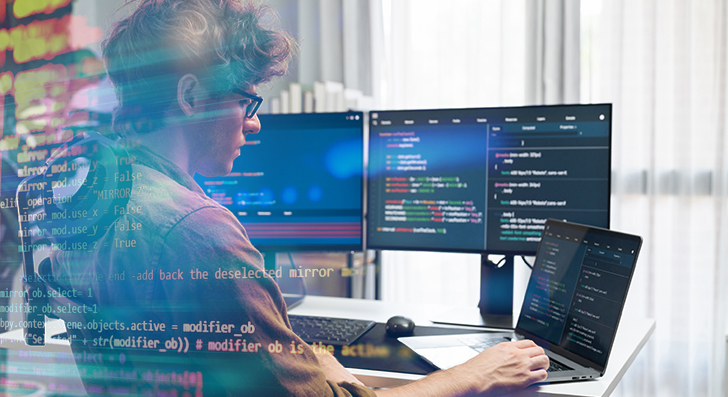
Scalability suggests your application can deal with development—extra people, far more info, and much more traffic—without breaking. For a developer, constructing with scalability in mind saves time and worry later on. Here’s a transparent and useful guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the Start
Scalability isn't really a little something you bolt on later on—it ought to be portion of your prepare from the beginning. A lot of apps fail every time they expand fast mainly because the original layout can’t handle the additional load. As a developer, you might want to Feel early regarding how your method will behave stressed.
Start by developing your architecture to generally be versatile. Stay clear of monolithic codebases the place everything is tightly linked. As a substitute, use modular design or microservices. These designs split your application into smaller, impartial areas. Each and every module or assistance can scale By itself with out impacting The full process.
Also, think about your database from day one particular. Will it require to deal with 1,000,000 people or simply just a hundred? Choose the correct sort—relational or NoSQL—based upon how your details will develop. Program for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
A further important stage is to prevent hardcoding assumptions. Don’t compose code that only performs less than current conditions. Consider what would occur In case your user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use design styles that aid scaling, like information queues or celebration-pushed programs. These support your app manage much more requests with no receiving overloaded.
If you Construct with scalability in mind, you are not just making ready for achievement—you are lowering long term headaches. A nicely-prepared process is simpler to maintain, adapt, and grow. It’s better to arrange early than to rebuild later on.
Use the proper Databases
Choosing the suitable database is really a key Component of constructing scalable applications. Not all databases are crafted the exact same, and using the wrong one can sluggish you down and even cause failures as your application grows.
Begin by understanding your knowledge. Is it remarkably structured, like rows within a desk? If yes, a relational databases like PostgreSQL or MySQL is an effective fit. These are definitely sturdy with relationships, transactions, and regularity. They also guidance scaling strategies like study replicas, indexing, and partitioning to take care of a lot more traffic and facts.
Should your details is more adaptable—like user action logs, products catalogs, or paperwork—think about a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured information and might scale horizontally more very easily.
Also, take into consideration your study and produce patterns. Will you be doing a lot of reads with much less writes? Use caching and skim replicas. Are you currently dealing with a significant write load? Explore databases which will deal with substantial generate throughput, or even occasion-dependent details storage systems like Apache Kafka (for short-term info streams).
It’s also clever to Imagine in advance. You may not need to have State-of-the-art scaling options now, but choosing a database that supports them indicates you won’t require to change later on.
Use indexing to speed up queries. Stay away from avoidable joins. Normalize or denormalize your data depending on your access patterns. And usually keep track of database overall performance as you develop.
In short, the proper databases is dependent upon your app’s construction, speed requirements, and how you expect it to grow. Choose time to select correctly—it’ll help save loads of hassle afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, just about every smaller delay adds up. Improperly penned code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s crucial that you Construct effective logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away something needless. Don’t choose the most advanced Resolution if a simple a person performs. Keep your capabilities limited, focused, and straightforward to test. Use profiling applications to uncover bottlenecks—spots exactly where your code usually takes way too lengthy to operate or makes use of too much memory.
Following, take a look at your databases queries. These frequently gradual factors down greater than the code alone. Make certain Just about every query only asks for the information you actually need to have. Avoid Decide on *, which fetches everything, and alternatively select unique fields. Use indexes to speed up lookups. And prevent performing too many joins, Primarily across massive tables.
If you recognize a similar information currently being asked for repeatedly, use caching. Retail store the outcomes briefly applying tools like Redis or Memcached and that means you don’t should repeat expensive operations.
Also, batch your database functions any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and will make your app much more productive.
Make sure to take a look at with significant datasets. Code and queries that function fantastic with one hundred data could crash every time they have to handle 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These ways website help your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it's to manage far more end users plus much more website traffic. If all the things goes as a result of a person server, it will eventually quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these equipment assistance keep the application quickly, stable, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of just one server executing every one of the perform, the load balancer routes customers to various servers based on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can send visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing data quickly so it may be reused quickly. When buyers ask for precisely the same info all over again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical sorts of caching:
one. Server-side caching (like Redis or Memcached) merchants information in memory for rapid access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents near the consumer.
Caching cuts down database load, increases speed, and will make your app additional effective.
Use caching for things which don’t change typically. And always be sure your cache is current when info does transform.
In short, load balancing and caching are basic but powerful equipment. Alongside one another, they help your application handle a lot more people, stay quickly, and Get well from problems. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To make scalable applications, you'll need equipment that permit your application mature effortlessly. That’s the place cloud platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain hardware or guess long term capability. When site visitors will increase, it is possible to incorporate far more methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you may scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection applications. You could deal with setting up your application as an alternative to controlling infrastructure.
Containers are Yet another important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it easy to maneuver your application amongst environments, out of your laptop to your cloud, with no surprises. Docker is the most well-liked tool for this.
Once your app utilizes various containers, instruments like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your app crashes, it restarts it immediately.
Containers also allow it to be straightforward to independent parts of your application into solutions. You could update or scale areas independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale fast, deploy quickly, and recover speedily when issues happen. If you need your application to expand without the need of limitations, start out utilizing these instruments early. They save time, minimize hazard, and enable you to keep centered on developing, not repairing.
Observe Every little thing
When you don’t monitor your application, you gained’t know when issues go Mistaken. Checking helps you see how your app is doing, location issues early, and make far better selections as your application grows. It’s a key A part of constructing scalable devices.
Start by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These show you how your servers and services are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—keep an eye on your application way too. Control how much time it will require for buyers to load internet pages, how frequently faults materialize, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Set up alerts for important problems. As an example, Should your response time goes over a limit or simply a company goes down, you'll want to get notified straight away. This can help you correct troubles quickly, usually prior to users even notice.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in errors or slowdowns, you can roll it again ahead of it leads to real problems.
As your app grows, traffic and details enhance. With out checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate equipment set up, you remain on top of things.
In a nutshell, monitoring will help you keep your application dependable and scalable. It’s not almost spotting failures—it’s about understanding your process and making sure it really works nicely, even stressed.
Final Feelings
Scalability isn’t only for huge providers. Even tiny applications want a solid foundation. By planning carefully, optimizing properly, and utilizing the correct instruments, you can Create applications that develop efficiently without breaking under pressure. Start out small, Feel major, and Create good.